BlockChyp Releases .NET / C# SDK
September 18, 2019
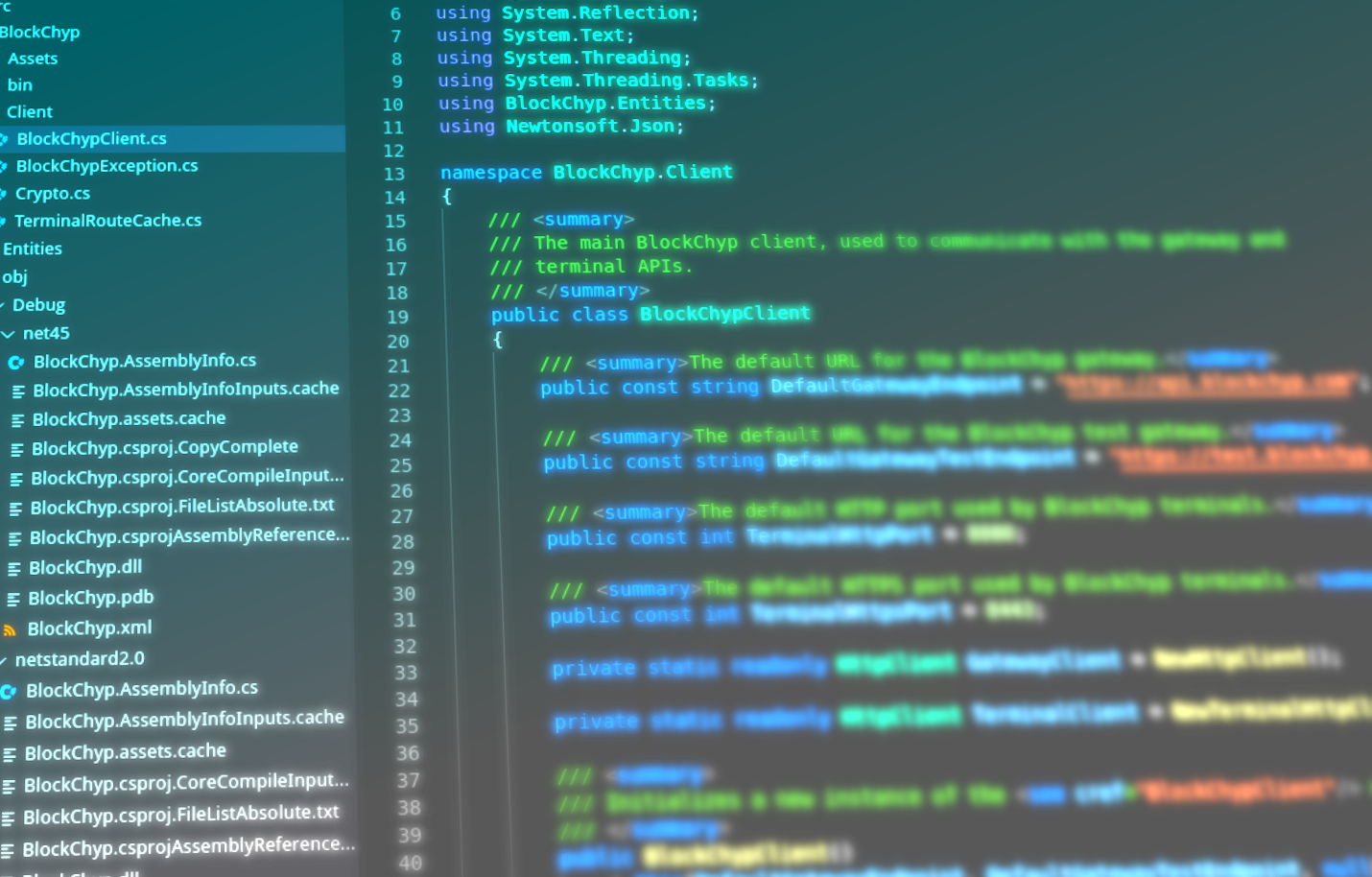
Today we are excited to announce the release of the official BlockChyp C# SDK . This new SDK allows developers in the Windows technology ecosystem to start running transactions on BlockChyp with just a few lines of code. Developers can use the SDK with any .NET Standard 2.0 or .NET Framework 4.5+ projects.
This new release adds binary level support for .NET and C# - the most popular technology platform for point-of-sale developers - to our existing list of supported SDK’s, which include Java, Go, and Javascript SDK’s.
Like all BlockChyp SDK’s, the C# SDK is fully open source with documentation and source code available on Github. For .NET developers using the Nuget package manager, BlockChyp has also been released on Nuget. No NDA or kludgey file downloads required.
Simple Integration
Getting up and running is easy. Just add the BlockChyp SDK to your project using the dotnet command line:
dotnet add package BlockChyp
Make sure you have a BlockChyp developer account, a test terminal, and a set of API Credentials. The code you’ll need to run your first transaction is as simple as this…
using System;
using BlockChyp.Client;
using BlockChyp.Entities;
namespace BlockChypSample
{
class Program
{
static void Main(string[] args)
{
// Be sure to use your test credentials here.
var credentials = new ApiCredentials(
"API_KEY",
"BEARER_TOKEN",
"SIGNING_KEY");
var blockchyp = new BlockChypClient(credentials);
var request = new AuthRequest
{
TerminalName = "Test Terminal",
Amount = "1.00",
};
AuthResponse response = blockchyp.Charge(request);
Console.WriteLine($"Transaction result: {response.ResponseDescription}");
}
}
}
Run the program and your terminal should wake up and prompt for a card. Insert or tap one of your BlockChyp test cards and you should receive a quick authorization response back from the gateway.
It’s that easy. For full documentation, start with the readme on Github.